This example contains the full workflow
- Request data from IRIS to make an MTH5
- Compute a transfer function using Aurora
- Adding the transfer function to MHT5
- Visualize using MTpy
Note: this example assumes that data availability (Network, Station, Channel, Start, End) are all previously known. If you do not know the data that you want to download use IRIS tools to get data availability.
1. Create MTH5 from IRIS¶
from pathlib import Path
import numpy as np
import pandas as pd
from mth5.mth5 import MTH5
from mth5.clients.make_mth5 import MakeMTH5
from matplotlib import pyplot as plt
%matplotlib inline
Set the path to save files to as the current working directory¶
default_path = Path().cwd()
print(default_path)
/home/jovyan/earthscope-mt-course/notebooks/mth5
Make the data inquiry as a DataFrame¶
There are a few ways to make the inquiry to request data.
- Make a DataFrame by hand. Here we will make a list of entries and then create a DataFrame with the proper column names
- You can create a CSV file with a row for each entry. There are some formatting that you need to be aware of. That is the column names and making sure that date-times are YYYY-MM-DDThh:mm:ss
Column Name | Description |
---|---|
network | FDSN Network code (2 letters) |
station | FDSN Station code (usually 5 characters) |
location | FDSN Location code (typically not used for MT) |
channel | FDSN Channel code (3 characters) |
start | Start time (YYYY-MM-DDThh:mm:ss) UTC |
end | End time (YYYY-MM-DDThh:mm:ss) UTC |
To create the request you can use any of the IRIS Data Services tools, a nice map view request GMAP. Be sure to identify 2 stations that recorded at the same time for at least a week. Network codes for MT that have correct metadata are ZU
and 8P
, other network codes like EM
still need work on making sure calibrations are correct.
channels = ["LFE", "LFN", "LFZ", "LQE", "LQN"]
WYYS2 = ["4P", "WYYS2", "2009-07-15T00:00:00", "2009-08-21T00:00:00"]
MTF20 = ["4P", "MTF20", "2009-07-03T00:00:00", "2009-08-13T00:00:00"]
request_list = []
for entry in [WYYS2, MTF20]:
for channel in channels:
request_list.append(
[entry[0], entry[1], "", channel, entry[2], entry[3]]
)
# Turn list into dataframe
request_df = pd.DataFrame(
request_list,
columns=[
"network",
"station",
"location",
"channel",
"start",
"end",
]
)
request_df.to_csv(default_path.joinpath("fdsn_request.csv"))
request_df
Make an MTH5¶
Now that we’ve created a request, and made sure that its what we expect, we can make an MTH5 file. The input can be either the DataFrame or the CSV file. We are going to time it just to get an indication how long it might take. Should take about 4 minutes.
Note: we are setting interact=False
. If you want to just to keep the file open to interogat it set interact=True
.
%%time
mth5_path = MakeMTH5.from_fdsn_client(request_df, interact=False)
print(f"Created {mth5_path}")
2024-10-17T12:04:04.365443-0700 | INFO | mth5.mth5 | _initialize_file | Initialized MTH5 0.2.0 file /home/jovyan/earthscope-mt-course/notebooks/mth5/4P_WYYS2_MTF20.h5 in mode w
2024-10-17T12:04:34.947556-0700 | INFO | mt_metadata.timeseries.filters.obspy_stages | create_filter_from_stage | Converting PoleZerosResponseStage electric_si_units to a CoefficientFilter.
2024-10-17T12:04:34.963637-0700 | INFO | mt_metadata.timeseries.filters.obspy_stages | create_filter_from_stage | Converting PoleZerosResponseStage electric_dipole_100.000 to a CoefficientFilter.
2024-10-17T12:04:35.050756-0700 | INFO | mt_metadata.timeseries.filters.obspy_stages | create_filter_from_stage | Converting PoleZerosResponseStage electric_si_units to a CoefficientFilter.
2024-10-17T12:04:35.066423-0700 | INFO | mt_metadata.timeseries.filters.obspy_stages | create_filter_from_stage | Converting PoleZerosResponseStage electric_dipole_100.000 to a CoefficientFilter.
2024-10-17T12:04:35.422952-0700 | INFO | mt_metadata.timeseries.filters.obspy_stages | create_filter_from_stage | Converting PoleZerosResponseStage electric_si_units to a CoefficientFilter.
2024-10-17T12:04:35.438127-0700 | INFO | mt_metadata.timeseries.filters.obspy_stages | create_filter_from_stage | Converting PoleZerosResponseStage electric_dipole_100.000 to a CoefficientFilter.
2024-10-17T12:04:35.518263-0700 | INFO | mt_metadata.timeseries.filters.obspy_stages | create_filter_from_stage | Converting PoleZerosResponseStage electric_si_units to a CoefficientFilter.
2024-10-17T12:04:35.533241-0700 | INFO | mt_metadata.timeseries.filters.obspy_stages | create_filter_from_stage | Converting PoleZerosResponseStage electric_dipole_100.000 to a CoefficientFilter.
2024-10-17T12:04:35.612481-0700 | INFO | mt_metadata.timeseries.filters.obspy_stages | create_filter_from_stage | Converting PoleZerosResponseStage electric_si_units to a CoefficientFilter.
2024-10-17T12:04:35.627792-0700 | INFO | mt_metadata.timeseries.filters.obspy_stages | create_filter_from_stage | Converting PoleZerosResponseStage electric_dipole_100.000 to a CoefficientFilter.
2024-10-17T12:04:35.708378-0700 | INFO | mt_metadata.timeseries.filters.obspy_stages | create_filter_from_stage | Converting PoleZerosResponseStage electric_si_units to a CoefficientFilter.
2024-10-17T12:04:35.723698-0700 | INFO | mt_metadata.timeseries.filters.obspy_stages | create_filter_from_stage | Converting PoleZerosResponseStage electric_dipole_100.000 to a CoefficientFilter.
2024-10-17T12:04:37.699187-0700 | INFO | mth5.groups.base | _add_group | RunGroup a already exists, returning existing group.
2024-10-17T12:04:38.103931-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id a. Setting to ch.run_metadata.id to a
2024-10-17T12:04:38.380238-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id a. Setting to ch.run_metadata.id to a
2024-10-17T12:04:38.654653-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id a. Setting to ch.run_metadata.id to a
2024-10-17T12:04:38.930692-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id a. Setting to ch.run_metadata.id to a
2024-10-17T12:04:39.210797-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id a. Setting to ch.run_metadata.id to a
2024-10-17T12:04:39.310401-0700 | INFO | mth5.groups.base | _add_group | RunGroup b already exists, returning existing group.
2024-10-17T12:04:40.902193-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id b. Setting to ch.run_metadata.id to b
2024-10-17T12:04:41.180722-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id b. Setting to ch.run_metadata.id to b
2024-10-17T12:04:41.458570-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id b. Setting to ch.run_metadata.id to b
2024-10-17T12:04:41.738640-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id b. Setting to ch.run_metadata.id to b
2024-10-17T12:04:42.021475-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id b. Setting to ch.run_metadata.id to b
2024-10-17T12:04:42.124787-0700 | INFO | mth5.groups.base | _add_group | RunGroup c already exists, returning existing group.
2024-10-17T12:04:43.344346-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id c. Setting to ch.run_metadata.id to c
2024-10-17T12:04:43.621538-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id c. Setting to ch.run_metadata.id to c
2024-10-17T12:04:43.896515-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id c. Setting to ch.run_metadata.id to c
2024-10-17T12:04:44.174169-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id c. Setting to ch.run_metadata.id to c
2024-10-17T12:04:44.454453-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id c. Setting to ch.run_metadata.id to c
2024-10-17T12:04:44.566038-0700 | INFO | mth5.groups.base | _add_group | RunGroup a already exists, returning existing group.
2024-10-17T12:04:44.972626-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id a. Setting to ch.run_metadata.id to a
2024-10-17T12:04:45.254814-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id a. Setting to ch.run_metadata.id to a
2024-10-17T12:04:45.529105-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id a. Setting to ch.run_metadata.id to a
2024-10-17T12:04:45.803441-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id a. Setting to ch.run_metadata.id to a
2024-10-17T12:04:46.078674-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id a. Setting to ch.run_metadata.id to a
2024-10-17T12:04:46.173180-0700 | INFO | mth5.groups.base | _add_group | RunGroup b already exists, returning existing group.
2024-10-17T12:04:48.159700-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id b. Setting to ch.run_metadata.id to b
2024-10-17T12:04:48.441483-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id b. Setting to ch.run_metadata.id to b
2024-10-17T12:04:48.724084-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id b. Setting to ch.run_metadata.id to b
2024-10-17T12:04:49.004931-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id b. Setting to ch.run_metadata.id to b
2024-10-17T12:04:49.289529-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id b. Setting to ch.run_metadata.id to b
2024-10-17T12:04:49.398657-0700 | INFO | mth5.groups.base | _add_group | RunGroup c already exists, returning existing group.
2024-10-17T12:04:50.252549-0700 | WARNING | mth5.timeseries.run_ts | validate_metadata | end time of dataset 2009-08-13T00:00:00+00:00 does not match metadata end 2009-08-13T17:15:39+00:00 updating metatdata value to 2009-08-13T00:00:00+00:00
2024-10-17T12:04:50.490357-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id c. Setting to ch.run_metadata.id to c
2024-10-17T12:04:50.771295-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id c. Setting to ch.run_metadata.id to c
2024-10-17T12:04:51.563312-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id c. Setting to ch.run_metadata.id to c
2024-10-17T12:04:51.840410-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id c. Setting to ch.run_metadata.id to c
2024-10-17T12:04:52.118128-0700 | WARNING | mth5.groups.run | from_runts | Channel run.id sr1_001 != group run.id c. Setting to ch.run_metadata.id to c
2024-10-17T12:04:52.524839-0700 | INFO | mth5.mth5 | close_mth5 | Flushing and closing /home/jovyan/earthscope-mt-course/notebooks/mth5/4P_WYYS2_MTF20.h5
2024-10-17T12:04:52.676235-0700 | WARNING | mth5.mth5 | filename | MTH5 file is not open or has not been created yet. Returning default name
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
File <timed exec>:3
NameError: name 'mth5_object' is not defined
If you are rerunning processing¶
mth5_path = Path("EM_WYYS2_MTF20.h5")
2. Estimate Transfer Function¶
Now that an MTH5 has been created we can estimate a transfer function using Aurora.
import warnings
from mtpy.processing import AuroraProcessing
warnings.filterwarnings('ignore')
Get a Run Summary¶
Get a run summary from the create mth5. We are going to provide the path to RunSummary
.
ap = AuroraProcessing()
ap.local_station_id = "WYYS2"
ap.local_mth5_path = mth5_path
ap.remote_station_id = "MTF20"
ap.remote_mth5_path = mth5_path
run_summary = ap.get_run_summary()
run_summary.mini_summary
Define a Kernel Dataset¶
Make a KernelDataset
to feed into Aurora. Here we are going to only use runs longer than 5000 seconds (80 minutes) to be sure we get good estimates at long periods.
Here you need to change the local station that you are going to process and the remote reference.
kernel_dataset = ap.create_kernel_dataset(
run_summary,
local_station_id=ap.local_station_id,
remote_station_id=ap.remote_station_id,
sample_rate=1,
)
kernel_dataset.drop_runs_shorter_than(5000)
kernel_dataset.mini_summary
Now define the processing Configuration¶
The only things we need to provide are our band processing scheme, and the data sample rate to generate a default processing configuration. The config is then told about the stations via the kernel dataset. Here we are passing it a band file so that we process longer periods.
Note: When doing only single station processing you need to specify RME processing (rather than remote reference processing which expects extra time series from another station)
config = ap.create_config(
kernel_dataset,
decimation_kwargs={"window.type": "hamming"},
**{"emtf_band_file":Path("../aurora/bs_256_29.cfg")},
)
processing_dict = {1:{"config":config, "kernel_dataset":kernel_dataset}}
Call process_mth5¶
%%time
processed_dict = ap.process(
[1],
processing_dict=processing_dict,
save_to_mth5=True,
)
mt_object = processed_dict[1]["tf"]
plot_res_phase = mt_object.plot_mt_response(plot_num=2)
2024-10-17T12:17:38.536853-0700 | INFO | aurora.pipelines.transfer_function_kernel | show_processing_summary | Processing Summary Dataframe:
2024-10-17T12:17:38.547677-0700 | INFO | aurora.pipelines.transfer_function_kernel | show_processing_summary |
duration has_data n_samples run station survey run_hdf5_reference station_hdf5_reference fc remote stft mth5_obj dec_level dec_factor sample_rate window_duration num_samples_window num_samples num_stft_windows
0 1367994.0 True 2400661 b MTF20 4P <HDF5 object reference> <HDF5 object reference> False True None HDF5 file is closed and cannot be accessed. 0 1.0 1.000000 128.0 128 1367994.0 21373.0
1 1367994.0 True 2400661 b MTF20 4P <HDF5 object reference> <HDF5 object reference> False True None HDF5 file is closed and cannot be accessed. 1 4.0 0.250000 512.0 128 341998.0 5342.0
2 1367994.0 True 2400661 b MTF20 4P <HDF5 object reference> <HDF5 object reference> False True None HDF5 file is closed and cannot be accessed. 2 4.0 0.062500 2048.0 128 85499.0 1334.0
3 1367994.0 True 2400661 b MTF20 4P <HDF5 object reference> <HDF5 object reference> False True None HDF5 file is closed and cannot be accessed. 3 4.0 0.015625 8192.0 128 21374.0 332.0
4 426132.0 True 1048354 c MTF20 4P <HDF5 object reference> <HDF5 object reference> False True None HDF5 file is closed and cannot be accessed. 0 1.0 1.000000 128.0 128 426132.0 6657.0
5 426132.0 True 1048354 c MTF20 4P <HDF5 object reference> <HDF5 object reference> False True None HDF5 file is closed and cannot be accessed. 1 4.0 0.250000 512.0 128 106533.0 1663.0
6 426132.0 True 1048354 c MTF20 4P <HDF5 object reference> <HDF5 object reference> False True None HDF5 file is closed and cannot be accessed. 2 4.0 0.062500 2048.0 128 26633.0 415.0
7 426132.0 True 1048354 c MTF20 4P <HDF5 object reference> <HDF5 object reference> False True None HDF5 file is closed and cannot be accessed. 3 4.0 0.015625 8192.0 128 6658.0 103.0
8 619809.0 True 1048354 c MTF20 4P <HDF5 object reference> <HDF5 object reference> False True None HDF5 file is closed and cannot be accessed. 0 1.0 1.000000 128.0 128 619809.0 9683.0
9 619809.0 True 1048354 c MTF20 4P <HDF5 object reference> <HDF5 object reference> False True None HDF5 file is closed and cannot be accessed. 1 4.0 0.250000 512.0 128 154952.0 2420.0
10 619809.0 True 1048354 c MTF20 4P <HDF5 object reference> <HDF5 object reference> False True None HDF5 file is closed and cannot be accessed. 2 4.0 0.062500 2048.0 128 38738.0 604.0
11 619809.0 True 1048354 c MTF20 4P <HDF5 object reference> <HDF5 object reference> False True None HDF5 file is closed and cannot be accessed. 3 4.0 0.015625 8192.0 128 9684.0 150.0
12 1367994.0 True 1798233 b WYYS2 4P <HDF5 object reference> <HDF5 object reference> False False None HDF5 file is closed and cannot be accessed. 0 1.0 1.000000 128.0 128 1367994.0 21373.0
13 1367994.0 True 1798233 b WYYS2 4P <HDF5 object reference> <HDF5 object reference> False False None HDF5 file is closed and cannot be accessed. 1 4.0 0.250000 512.0 128 341998.0 5342.0
14 1367994.0 True 1798233 b WYYS2 4P <HDF5 object reference> <HDF5 object reference> False False None HDF5 file is closed and cannot be accessed. 2 4.0 0.062500 2048.0 128 85499.0 1334.0
15 1367994.0 True 1798233 b WYYS2 4P <HDF5 object reference> <HDF5 object reference> False False None HDF5 file is closed and cannot be accessed. 3 4.0 0.015625 8192.0 128 21374.0 332.0
16 426132.0 True 1798233 b WYYS2 4P <HDF5 object reference> <HDF5 object reference> False False None HDF5 file is closed and cannot be accessed. 0 1.0 1.000000 128.0 128 426132.0 6657.0
17 426132.0 True 1798233 b WYYS2 4P <HDF5 object reference> <HDF5 object reference> False False None HDF5 file is closed and cannot be accessed. 1 4.0 0.250000 512.0 128 106533.0 1663.0
18 426132.0 True 1798233 b WYYS2 4P <HDF5 object reference> <HDF5 object reference> False False None HDF5 file is closed and cannot be accessed. 2 4.0 0.062500 2048.0 128 26633.0 415.0
19 426132.0 True 1798233 b WYYS2 4P <HDF5 object reference> <HDF5 object reference> False False None HDF5 file is closed and cannot be accessed. 3 4.0 0.015625 8192.0 128 6658.0 103.0
20 619809.0 True 1225636 c WYYS2 4P <HDF5 object reference> <HDF5 object reference> False False None HDF5 file is closed and cannot be accessed. 0 1.0 1.000000 128.0 128 619809.0 9683.0
21 619809.0 True 1225636 c WYYS2 4P <HDF5 object reference> <HDF5 object reference> False False None HDF5 file is closed and cannot be accessed. 1 4.0 0.250000 512.0 128 154952.0 2420.0
22 619809.0 True 1225636 c WYYS2 4P <HDF5 object reference> <HDF5 object reference> False False None HDF5 file is closed and cannot be accessed. 2 4.0 0.062500 2048.0 128 38738.0 604.0
23 619809.0 True 1225636 c WYYS2 4P <HDF5 object reference> <HDF5 object reference> False False None HDF5 file is closed and cannot be accessed. 3 4.0 0.015625 8192.0 128 9684.0 150.0
2024-10-17T12:17:38.549452-0700 | INFO | aurora.pipelines.transfer_function_kernel | memory_check | Total memory: 30.89 GB
2024-10-17T12:17:38.550261-0700 | INFO | aurora.pipelines.transfer_function_kernel | memory_check | Total Bytes of Raw Data: 0.036 GB
2024-10-17T12:17:38.551120-0700 | INFO | aurora.pipelines.transfer_function_kernel | memory_check | Raw Data will use: 0.116 % of memory
2024-10-17T12:17:38.575596-0700 | INFO | aurora.pipelines.process_mth5 | process_mth5_legacy | Processing config indicates 4 decimation levels
2024-10-17T12:17:38.577525-0700 | INFO | aurora.pipelines.transfer_function_kernel | valid_decimations | After validation there are 4 valid decimation levels
2024-10-17T12:17:41.040461-0700 | WARNING | mth5.timeseries.run_ts | validate_metadata | end time of dataset 2009-07-31T19:39:01+00:00 does not match metadata end 2009-08-05T19:09:39+00:00 updating metatdata value to 2009-07-31T19:39:01+00:00
2024-10-17T12:17:43.544184-0700 | WARNING | mth5.timeseries.run_ts | validate_metadata | start time of dataset 2009-07-15T23:39:07+00:00 does not match metadata start 2009-07-04T00:48:01+00:00 updating metatdata value to 2009-07-15T23:39:07+00:00
2024-10-17T12:17:44.672510-0700 | WARNING | mth5.timeseries.run_ts | validate_metadata | start time of dataset 2009-07-31T20:47:27+00:00 does not match metadata start 2009-07-15T23:39:07+00:00 updating metatdata value to 2009-07-31T20:47:27+00:00
2024-10-17T12:17:45.799513-0700 | WARNING | mth5.timeseries.run_ts | validate_metadata | end time of dataset 2009-08-05T19:09:39+00:00 does not match metadata end 2009-08-13T00:00:00+00:00 updating metatdata value to 2009-08-05T19:09:39+00:00
2024-10-17T12:17:47.149605-0700 | WARNING | mth5.timeseries.run_ts | validate_metadata | end time of dataset 2009-08-13T00:00:00+00:00 does not match metadata end 2009-08-20T00:17:06+00:00 updating metatdata value to 2009-08-13T00:00:00+00:00
2024-10-17T12:17:48.495199-0700 | WARNING | mth5.timeseries.run_ts | validate_metadata | start time of dataset 2009-08-05T19:49:51+00:00 does not match metadata start 2009-07-31T20:47:27+00:00 updating metatdata value to 2009-08-05T19:49:51+00:00
2024-10-17T12:17:48.519318-0700 | INFO | mtpy.processing.kernel_dataset | initialize_dataframe_for_processing | Dataset dataframe initialized successfully
2024-10-17T12:17:48.520248-0700 | INFO | aurora.pipelines.transfer_function_kernel | update_dataset_df | Dataset Dataframe Updated for decimation level 0 Successfully
2024-10-17T12:17:50.105458-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:17:51.756430-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:17:52.486133-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:17:53.260272-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:17:54.145650-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:17:55.069230-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:17:55.223189-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 25.728968s (0.038867Hz)
2024-10-17T12:17:55.668992-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 19.929573s (0.050177Hz)
2024-10-17T12:17:56.380567-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 15.164131s (0.065945Hz)
2024-10-17T12:17:56.996077-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 11.746086s (0.085135Hz)
2024-10-17T12:17:57.762430-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 9.195791s (0.108745Hz)
2024-10-17T12:17:58.519232-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 7.362526s (0.135823Hz)
2024-10-17T12:17:59.457081-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 5.856115s (0.170762Hz)
2024-10-17T12:18:00.567262-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 4.682492s (0.213562Hz)
2024-10-17T12:18:01.890887-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 3.784813s (0.264214Hz)
2024-10-17T12:18:03.377118-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 3.025193s (0.330557Hz)
2024-10-17T12:18:05.115179-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 2.519631s (0.396883Hz)
2024-10-17T12:18:07.385257-0700 | INFO | aurora.pipelines.transfer_function_kernel | update_dataset_df | DECIMATION LEVEL 1
2024-10-17T12:18:08.005255-0700 | INFO | aurora.pipelines.transfer_function_kernel | update_dataset_df | Dataset Dataframe Updated for decimation level 1 Successfully
2024-10-17T12:18:08.646510-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:09.322952-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:09.781734-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:10.291245-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:10.786181-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:11.326719-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:11.365367-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 102.915872s (0.009717Hz)
2024-10-17T12:18:11.534030-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 85.631182s (0.011678Hz)
2024-10-17T12:18:11.729172-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 68.881694s (0.014518Hz)
2024-10-17T12:18:11.968225-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 54.195827s (0.018452Hz)
2024-10-17T12:18:12.218745-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 43.003958s (0.023254Hz)
2024-10-17T12:18:12.542687-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 33.310722s (0.030020Hz)
2024-10-17T12:18:13.089944-0700 | INFO | aurora.pipelines.transfer_function_kernel | update_dataset_df | DECIMATION LEVEL 2
2024-10-17T12:18:13.300320-0700 | INFO | aurora.pipelines.transfer_function_kernel | update_dataset_df | Dataset Dataframe Updated for decimation level 2 Successfully
2024-10-17T12:18:13.745345-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:14.237428-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:14.638498-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:15.088761-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:15.502496-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:15.960355-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:15.980930-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 411.663489s (0.002429Hz)
2024-10-17T12:18:16.080575-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 342.524727s (0.002919Hz)
2024-10-17T12:18:16.197946-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 275.526776s (0.003629Hz)
2024-10-17T12:18:16.335137-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 216.783308s (0.004613Hz)
2024-10-17T12:18:16.472314-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 172.015831s (0.005813Hz)
2024-10-17T12:18:16.635186-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 133.242890s (0.007505Hz)
2024-10-17T12:18:16.989268-0700 | INFO | aurora.pipelines.transfer_function_kernel | update_dataset_df | DECIMATION LEVEL 3
2024-10-17T12:18:17.096757-0700 | INFO | aurora.pipelines.transfer_function_kernel | update_dataset_df | Dataset Dataframe Updated for decimation level 3 Successfully
2024-10-17T12:18:17.500033-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:17.950631-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:18.341954-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:18.779947-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:19.175960-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:19.614262-0700 | INFO | aurora.pipelines.process_mth5 | save_fourier_coefficients | Skip saving FCs. dec_level_config.save_fc = False
2024-10-17T12:18:19.630678-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 2064.189975s (0.000484Hz)
2024-10-17T12:18:19.706317-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 1514.701336s (0.000660Hz)
2024-10-17T12:18:19.783053-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 1042.488956s (0.000959Hz)
2024-10-17T12:18:19.864218-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 723.371271s (0.001382Hz)
2024-10-17T12:18:20.136700-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 532.971560s (0.001876Hz)
2024-10-17T12:18:20.252673-0700 | INFO | aurora.time_series.frequency_band_helpers | get_band_for_tf_estimate | Processing band 412.837995s (0.002422Hz)
2024-10-17T12:18:20.644786-0700 | INFO | mth5.mth5 | close_mth5 | Flushing and closing /home/jovyan/earthscope-mt-course/notebooks/mth5/4P_WYYS2_MTF20.h5
2024-10-17T12:18:20.645940-0700 | INFO | mth5.mth5 | close_mth5 | Flushing and closing /home/jovyan/earthscope-mt-course/notebooks/mth5/4P_WYYS2_MTF20.h5
2024-10-17T12:18:21.380331-0700 | INFO | mth5.mth5 | close_mth5 | Flushing and closing /home/jovyan/earthscope-mt-course/notebooks/mth5/4P_WYYS2_MTF20.h5
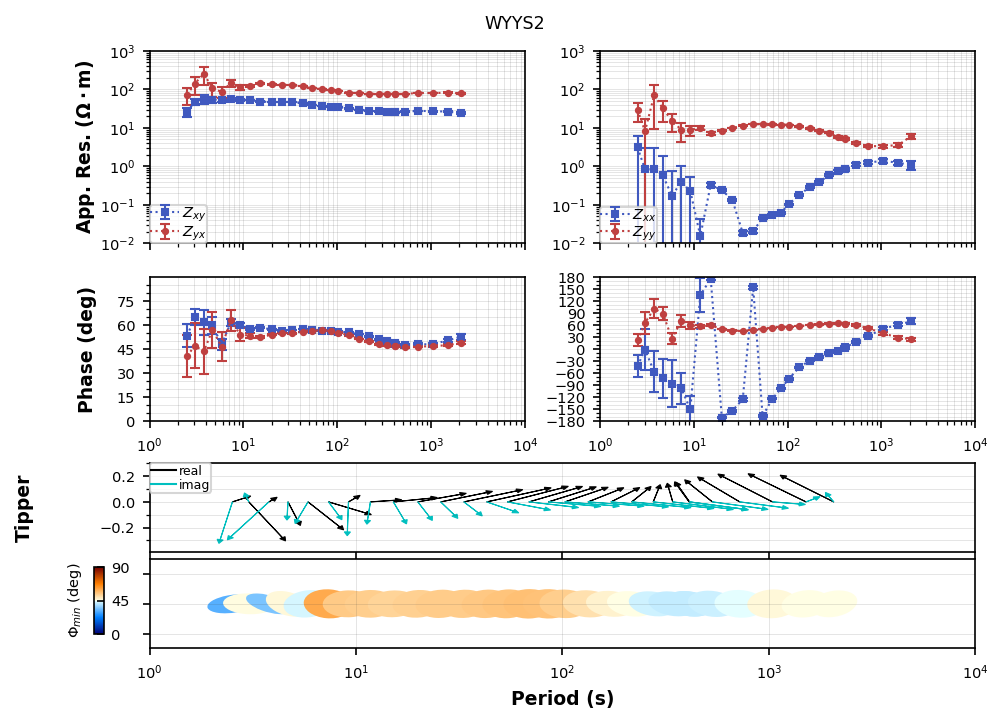
CPU times: user 1min 20s, sys: 1.26 s, total: 1min 21s
Wall time: 45.3 s
Write Transfer Function to a File¶
Here we will write to an EMTF XML format
xml_file_base = f"example_tf.xml"
emtf_obj = mt_object.write(fn=xml_file_base, file_type="emtfxml")
Plot Phase Tensor¶
plot_pt = mt_object.plot_phase_tensor(fig_num=6)
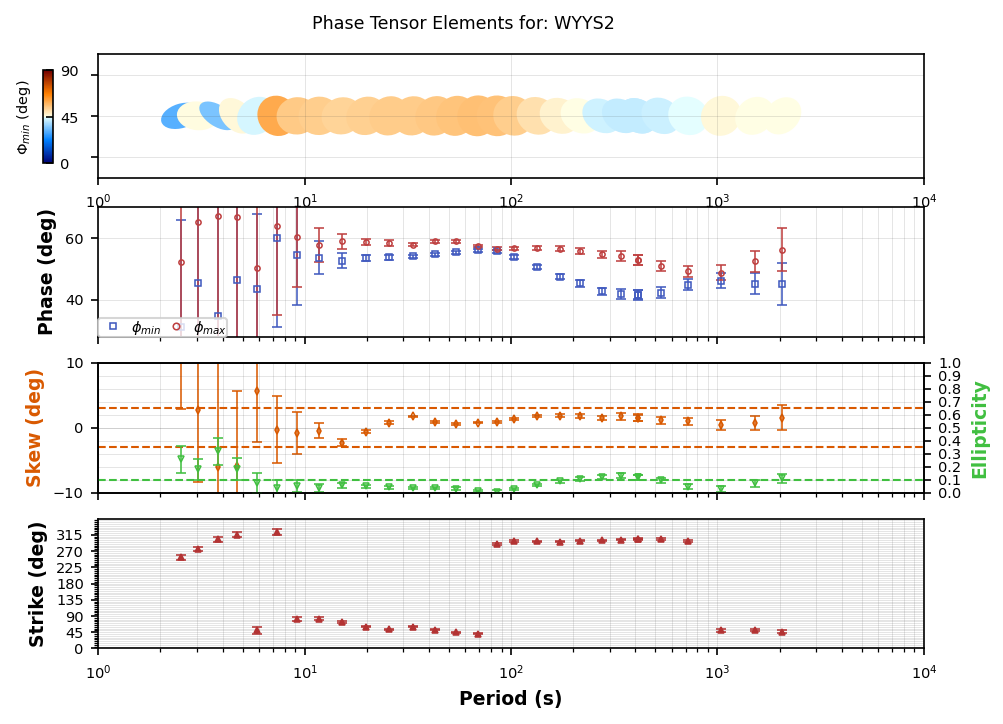